Index
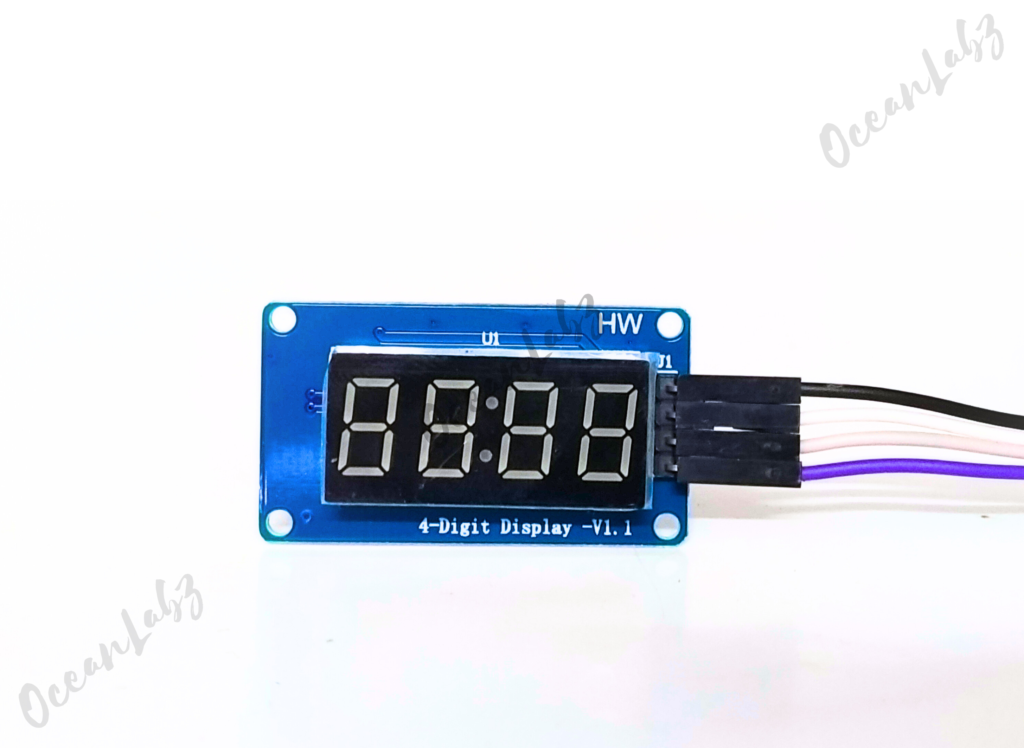
Introduction
The 4-Digit 7-Segment Display Module with the TM1637 driver IC is a complete and user-friendly module designed to simplify the integration of multiple 7-segment displays with an Arduino. Unlike standalone displays, this module features four digits pre-mounted on a compact board, making it perfect for easily displaying numerical values such as time, temperature, or other data in a clear and readable format.
The TM1637 IC embedded in the module allows for easy control using just two pins for data communication, which significantly reduces the complexity of wiring and programming. In this tutorial, we will learn how to connect the TM1637 4-digit display module to an Arduino, write code to control the display, and explore various applications for this versatile module, enhancing your ability to present data visually in your electronic projects.
Required Components
- Arduino UNO, Nano
- 4-Digit 7-Segment Display module (TM1637-based)
- Jumper wires
- Breadboard
Pinout

Circuit Diagram / Wiring
- VCC (TM1637) → 5V (Arduino)
- GND (TM1637) → GND (Arduino)
- DIO (TM1637) → Pin 2 (Arduino)
- CLK (TM1637) → Pin 3 (Arduino)
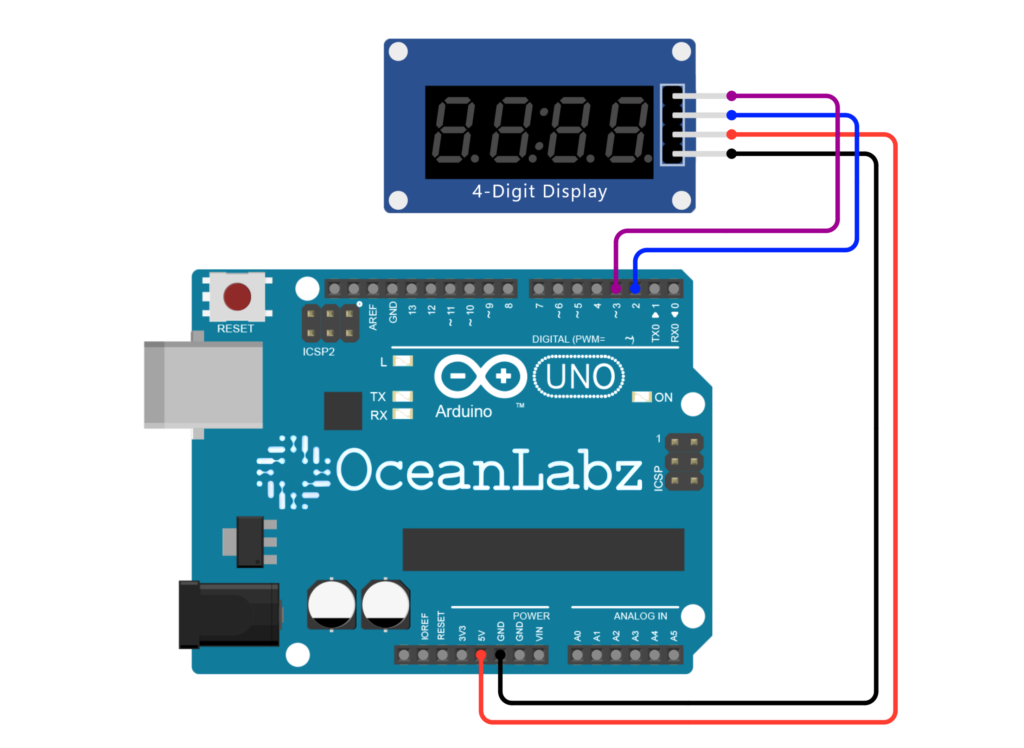
Arduino Code / Programming
- Go to the “Libraries” tab on the left-hand side of the screen.
- Click on the “Library Manager” button (book icon) at the top of the Libraries tab.
- In the Library Manager window, type “TM1637” in the search bar.
- Locate the “TM1637” library click on the “Install” button next to it.
- Wait for the library to be installed, and you’re ready to use the TM1637 library in your projects.
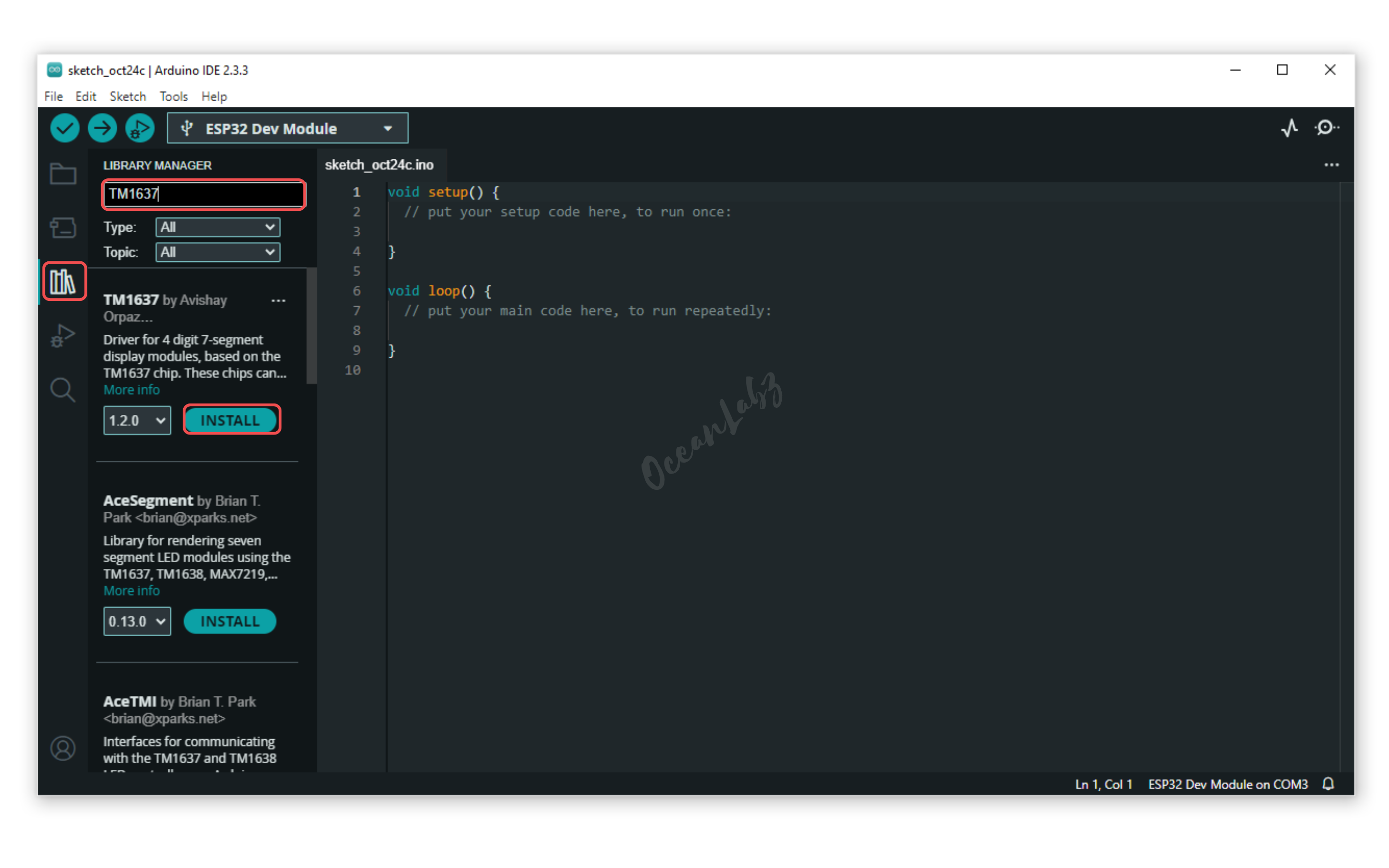
- Use the following code
#include <TM1637Display.h>
// Define the connection pins for the display
#define CLK 3
#define DIO 2
// Create an instance of the TM1637Display library
TM1637Display display(CLK, DIO);
// Set the countdown time in seconds
int countdownTime = 180; // 3 minutes (180 seconds)
void setup() {
// Set the brightness of the display (0-7)
display.setBrightness(5);
}
void loop() {
// If the countdown has ended, show "0000" and stop the timer
if (countdownTime <= 0) {
display.showNumberDec(0, true);
return; // Stop the timer
}
// Calculate minutes and seconds
int minutes = countdownTime / 60;
int seconds = countdownTime % 60;
// Format the time as MM:SS
int timeToShow = (minutes * 100) + seconds;
// Display the time
display.showNumberDecEx(timeToShow, 0b01000000, true); // The 0b01000000 lights up the colon
// Wait for 0.1 seconds (very fast countdown)
delay(100);
// Decrement the countdown time
countdownTime--;
}
Explanation of the Code
The code creates a fast countdown timer starting from 3 minutes (180 seconds) using a TM1637 4-digit display. It displays the remaining time in minutes and seconds (MMformat) with a colon between them. The countdown updates every 0.1 seconds, and once the time reaches zero, it shows “0000” and stops.
Arduino project – Creating a clock with TM1637Display and DS3231 (RTC)
Here’s how you can create a clock using the TM1637 4-Digit Display and the DS3231 RTC module with Arduino
Circuit Diagram / Wiring
Arduino Code
#include <Wire.h>
#include <RTClib.h>
#include <TM1637Display.h>
// DS3231 RTC setup
RTC_DS3231 rtc;
// TM1637 setup
#define CLK 2
#define DIO 3
TM1637Display display(CLK, DIO);
void setup() {
Wire.begin();
display.setBrightness(7); // Set display brightness (0-7)
if (!rtc.begin()) {
Serial.println("RTC not found!");
while (1);
}
if (rtc.lostPower()) {
rtc.adjust(DateTime(F(__DATE__), F(__TIME__))); // Set time from compile time
}
}
void loop() {
DateTime now = rtc.now(); // Get current time
// Display time in HH:MM format
int hour = now.hour();
int minute = now.minute();
display.showNumberDecEx(hour * 100 + minute, 0b01000000, true);
delay(1000); // Update every second
}
Explanation
- RTC Module: Provides real-time clock data with accurate timekeeping.
- TM1637 Display: Shows the current time in
HH:MM
format with a colon separator. - I2C Communication: Used to fetch time from DS3231 to display on TM1637.
Challenges
Challenge 1: Precision Countdown Game
Problem Statement:
- This game begins with the display counting up from 0000, and the goal is for the player to press the button as close to 9999 as possible.
- If the player successfully presses the button at exactly 9999, they win, and the display briefly flashes “WIN.”
- If they miss the mark (e.g., pressing at any number other than 9999), the display shows “LOSE” before resetting to try again.
Code:
#include <TM1637Display.h>
const int CLK = 2;
const int DIO = 3;
const int switchPin = 4;
TM1637Display display(CLK, DIO);
int count = 0;
bool gameActive = true;
void setup() {
pinMode(switchPin, INPUT);
display.setBrightness(0x0f);
display.showNumberDec(count, true); // Initialize display at "0000"
}
void loop() {
if (gameActive) {
display.showNumberDec(count, true, 4); // Display current count
// Increase the count every 100 milliseconds
delay(100);
count++;
// Check for button press to try to win
if (digitalRead(switchPin) == HIGH) {
delay(20); // Debounce
if (count == 9999) {
showWin();
} else {
showLose();
}
}
// Reset count if it reaches 9999 without a button press
if (count > 9999) {
count = 0; // Reset to start the game over
}
}
}
void showWin() {
display.showNumberDecEx(9999, 0b01000000, true); // Flash "WIN"
delay(2000); // Show for 2 seconds
resetGame();
}
void showLose() {
display.showNumberDecEx(8888, 0b01000000, true); // Flash "LOSE" (use "8888")
delay(2000); // Show for 2 seconds
resetGame();
}
void resetGame() {
count = 0;
gameActive = true;
display.showNumberDec(count, true);
}
Challenge 2: Countdown Timer with Adjustable Duration
Problem Statement:
- The display shows a countdown in seconds.
- Each time the button is pressed, it adds 5 seconds to the timer (up to a maximum of 60 seconds).
- Holding the button for 3 seconds starts the countdown.
- When the countdown reaches zero, the display flashes “0000” until reset.
Code:
#include <TM1637Display.h>
const int CLK = 2;
const int DIO = 3;
const int switchPin = 4;
TM1637Display display(CLK, DIO);
int countdownTime = 0;
bool counting = false;
unsigned long lastButtonPress = 0;
void setup() {
pinMode(switchPin, INPUT);
display.setBrightness(0x0f);
display.showNumberDec(countdownTime, true);
}
void loop() {
if (digitalRead(switchPin) == HIGH) {
if (!counting) {
countdownTime += 5;
if (countdownTime > 60) countdownTime = 60; // Limit to 60 seconds
display.showNumberDec(countdownTime, true);
delay(500); // Debounce
} else {
lastButtonPress = millis();
}
}
if (digitalRead(switchPin) == HIGH && millis() - lastButtonPress >= 3000) {
counting = true;
lastButtonPress = millis();
}
if (counting && countdownTime > 0) {
display.showNumberDec(countdownTime, false, 4);
delay(1000);
countdownTime--;
}
if (countdownTime == 0 && counting) {
flashDisplay();
counting = false;
}
}
void flashDisplay() {
for (int i = 0; i < 10; i++) {
display.showNumberDecEx(0, 0b11111111); // Display "0000"
delay(250);
display.clear();
delay(250);
}
}
Testing and Troubleshooting
- If the display does not light up, check all connections.
- Make sure the correct pins are used for
CLK
andDIO
. - Use the Serial Monitor to debug the code and test values.