Index
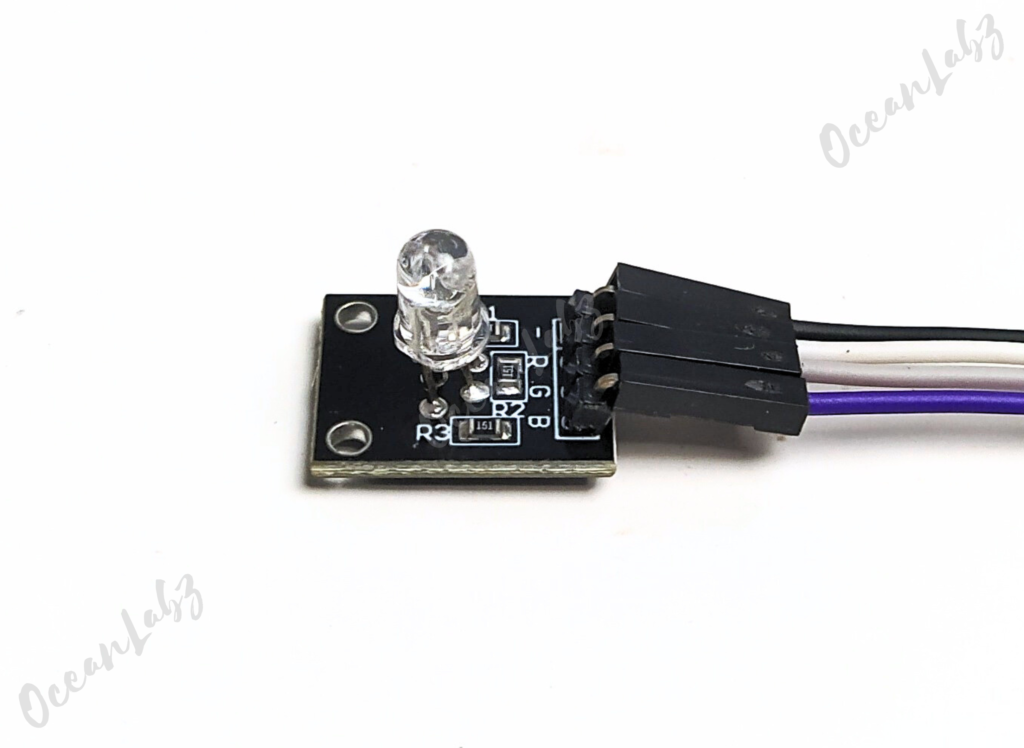
Introduction
The KY-016 RGB module allows you to produce a variety of colors by mixing the red, green, and blue lights. It has built-in resistors for each color, making it easy to connect directly to the Arduino.
Required Components
- Arduino UNO R3 SMD
- KY-016 RGB LED module
- Jumper wires
- Breadboard (optional)
Pinout

Circuit Diagram / Wiring
- RGB (GND) → Arduino GND
- RGB (RED) → Arduino D9
- RGB (GREEN) → Arduino D10
- RGB (BLUE) → Arduino D11

Arduino Code / Programming
The following code shows how to control the KY-016 RGB module to produce different colors using the analogWrite()
function.
int redPin = 9; // Pin connected to the red LED
int greenPin = 10; // Pin connected to the green LED
int bluePin = 11; // Pin connected to the blue LED
void setup() {
// Set the RGB LED pins as outputs
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
}
void loop() {
// Set the LED to red
setColor(0, 255, 255); // Red ON, Green and Blue OFF
delay(1000); // Wait for 1 second
// Set the LED to green
setColor(255, 0, 255); // Green ON, Red and Blue OFF
delay(1000); // Wait for 1 second
// Set the LED to blue
setColor(255, 255, 0); // Blue ON, Red and Green OFF
delay(1000); // Wait for 1 second
// Set the LED to yellow
setColor(0, 0, 255); // Red and Green ON, Blue OFF
delay(1000); // Wait for 1 second
// Set the LED to cyan
setColor(255, 0, 0); // Green and Blue ON, Red OFF
delay(1000); // Wait for 1 second
// Set the LED to magenta
setColor(0, 255, 0); // Red and Blue ON, Green OFF
delay(1000); // Wait for 1 second
// Turn off the LED
setColor(255, 255, 255);
delay(1000); // Wait for 1 second
}
// Function to set the color of the RGB LED
void setColor(int redValue, int greenValue, int blueValue) {
analogWrite(redPin, redValue);
analogWrite(greenPin, greenValue);
analogWrite(bluePin, blueValue);
}
Explanation of the Code
- Pin Definitions: The pins for the red, green, and blue LEDs are defined.
- Setup Function: Sets the RGB LED pins as outputs.
- Loop Function: Changes the color of the RGB LED by calling the
setColor()
function with different values. - setColor() Function: Uses the
analogWrite()
function to set the brightness of each LED.
Testing and Troubleshooting
- Check Connections: Ensure the connections
- Adjust Colors: You can fine-tune the RGB values in
setColor()
for different colors.