Index

Introduction
This tutorial guides you through using an ultrasonic sonar sensor with Arduino to measure distances. Ultrasonic sensors work by emitting sound waves and measuring the time it takes for the echo to return, allowing you to detect obstacles or measure distances accurately.
Required Components
- Arduino Board (e.g., Arduino Uno)
- Ultrasonic sensor (e.g., HC-SR04)
- Jumper Wires
- Breadboard (optional)
Pinout
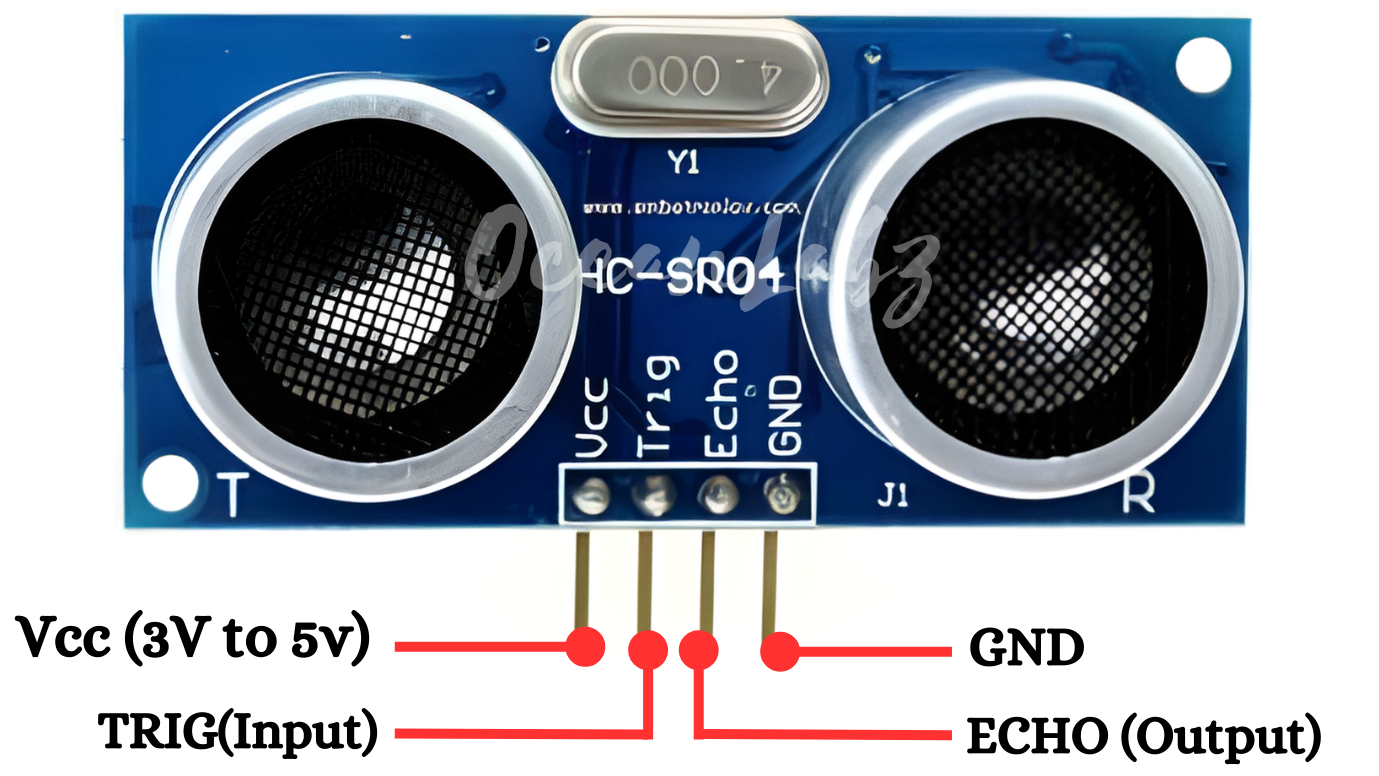
Circuit Diagram / Wiring
- ULTRASONIC SENSOR VCC → 5V (Arduino)
- ULTRASONIC SENSOR GND → GND (Arduino)
- ULTRASONIC SENSOR TRIG → Pin D8 (Arduino)
- ULTRASONIC SENSOR ECHO → Pin D9 (Arduino)

Programming With Arduino
- Go to the “Libraries” tab on the left-hand side of the screen.
- Click on the “Library Manager” button (book icon) at the top of the Libraries tab.
- In the Library Manager window, type “NewPing” in the search bar, locate the NewPing library, and click on the “Install” button next to it.

- Copy the provided code into your Arduino IDE.
#include <NewPing.h>
#define TRIGGER_PIN 8 // Pin for the trigger
#define ECHO_PIN 9 // Pin for the echo
#define MAX_DISTANCE 200 // Maximum distance to measure in cm
NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE);
void setup() {
Serial.begin(9600); // Initialize serial communication
}
void loop() {
delay(50); // Wait for a short duration
unsigned int distance = sonar.ping_cm(); // Measure distance
Serial.print("Distance: "); // Print the distance
Serial.print(distance);
Serial.println(" cm");
}
Explanation
- The code uses an ultrasonic sensor to measure distance in centimeters.
TRIGGER_PIN
sends sound pulses, andECHO_PIN
receives the returning signal.- The
NewPing
library calculates the distance based on the time taken for the pulse to return. - The distance is printed to the Serial Monitor every 50 milliseconds for real-time updates.
Testing and Troubleshooting
- Ensure proper connections of the ultrasonic sensor to the Arduino.
- Open the Serial Monitor to view distance measurements.
- If the readings are inconsistent, check for proper wiring and ensure the sensor is pointed toward an object.