Index
Introduction
This project uses an HC-SR04 ultrasonic sensor and a WS2812 NeoPixel LED strip to visualize distance measurements. The LEDs dynamically light up based on the distance detected by the sonar sensor. Each LED represents a specific range of the measured distance. The closer the object, the fewer LEDs light up, creating a real-time visual feedback system. Perfect for interactive and educational projects!
Required Components
- Arduino UNO / Nano
- Ultrasonic Sonar Sensor
- WS2812B LED strip
- Jumper wire
- Bread Board
Circuit Diagram / Wiring
- Sonar Sensor With Arduino
- Sonar Sensor VCC → 5V (Arduino)
- Sonar Sensor GND → GND (Arduino)
- Sonar Sensor TRIG→ Pin 9 (Arduino)
- Sonar Sensor ECHO→ Pin 10 (Arduino)
- WS2812B With Arduino
- WS2812B VCC → 5V (Arduino)
- WS2812B GND → GND (Arduino)
- WS2812B DO→ Pin 6 (Arduino)
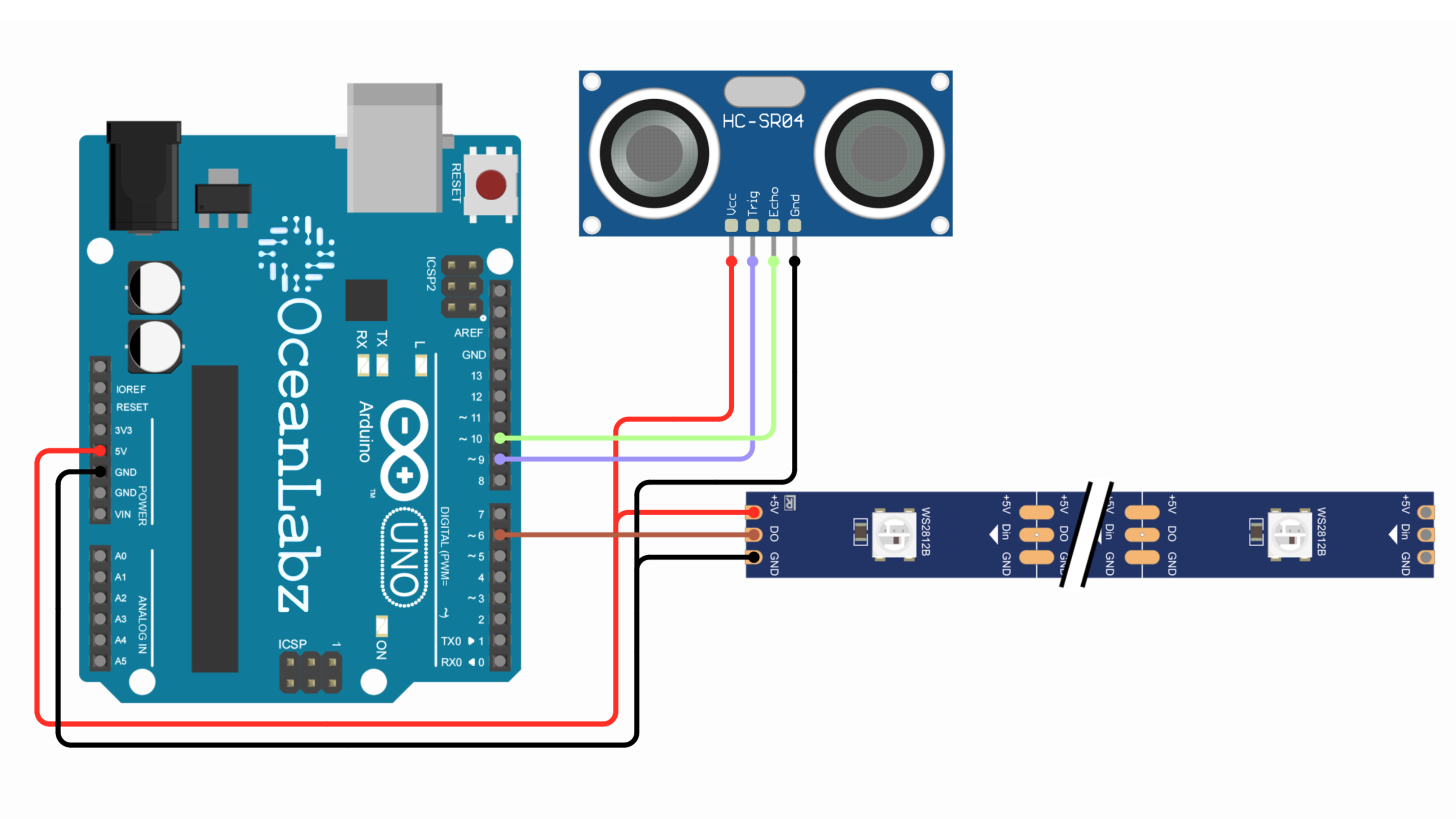
Arduino Code / Programming
Make sure you have the required libraries installed:
- Adafruit NeoPixel library for WS2812B LED Strip
- HCSR04 library for Ultrasonic sensor
#include <Adafruit_NeoPixel.h>
#include <HCSR04.h>
#define PIN 6 // Which pin on the Arduino is connected to the NeoPixels?
#define NUMPIXELS 29 // How many NeoPixels are attached to the Arduino?
Adafruit_NeoPixel pixels(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800);
UltraSonicDistanceSensor distanceSensor(9, 10); // Initialize sensor that uses digital pins 9 and 10.
int current_Last_LED=0;
void setup()
{
pixels.begin();
}
void loop()
{
pixels.clear(); // Set all pixel colors to 'off'
current_Last_LED=distanceSensor.measureDistanceCm()*0.8;
for(int i=0; i<current_Last_LED; i++) // For each pixel in strip...
{
pixels.setPixelColor(i, 0,0,255);
}
pixels.show(); // Send the updated pixel colors to the hardware.
}
Explanation
- Distance Measurement: The HC-SR04 ultrasonic sensor measures the distance to an object in centimeters.
- LED Control: The measured distance is used to light up a portion of the WS2812 LED strip, where each LED represents a segment of the distance.
- Dynamic Feedback: The closer the object, the more LEDs light up in blue; the farther the object, fewer LEDs light up.
- Visualization: The LED strip provides a real-time visual representation of the proximity of objects based on the distance sensor’s output.