Index
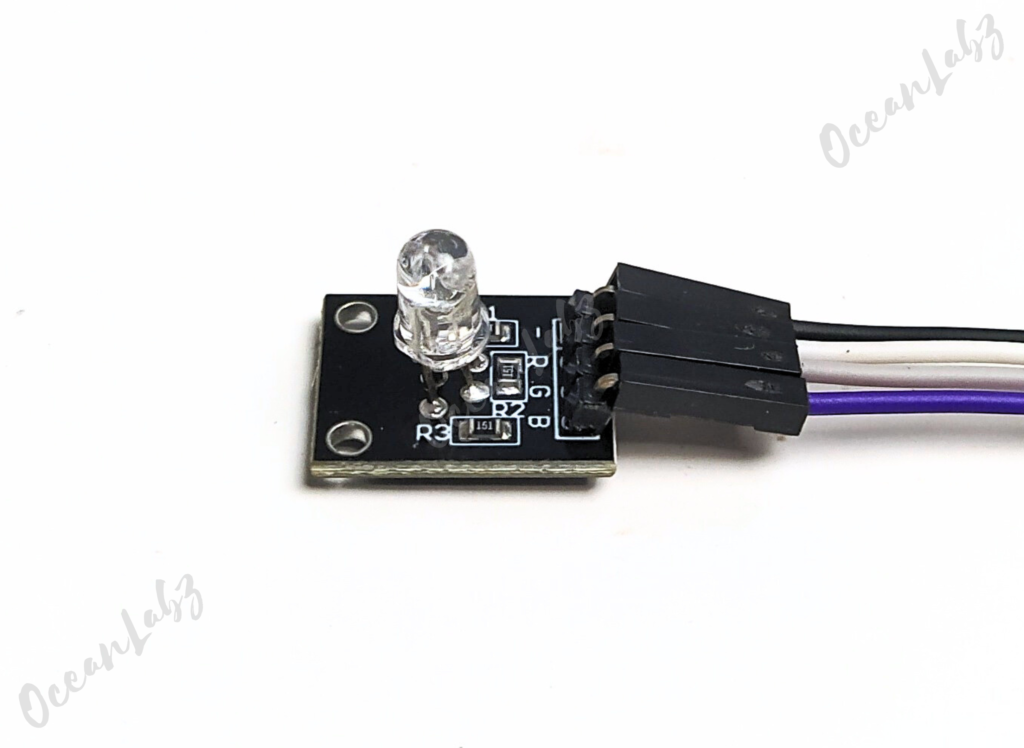
Introduction
The KY-016 RGB module allows you to produce a variety of colors by mixing the red, green, and blue lights. It has built-in resistors for each color, making it easy to connect directly to the Arduino.
Required Components
- Arduino UNO R3 SMD
- KY-016 RGB LED module
- Jumper wires
- Breadboard (optional)
Pinout

Circuit Diagram / Wiring
- RGB (GND) → Arduino GND
- RGB (RED) → Arduino D9
- RGB (GREEN) → Arduino D10
- RGB (BLUE) → Arduino D11

Arduino Code / Programming
The following code shows how to control the KY-016 RGB module to produce different colors using the analogWrite()
function.
int redPin = 9; // Pin connected to the red LED
int greenPin = 10; // Pin connected to the green LED
int bluePin = 11; // Pin connected to the blue LED
void setup() {
// Set the RGB LED pins as outputs
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
}
void loop() {
// Set the LED to red
setColor(0, 255, 255); // Red ON, Green and Blue OFF
delay(1000); // Wait for 1 second
// Set the LED to green
setColor(255, 0, 255); // Green ON, Red and Blue OFF
delay(1000); // Wait for 1 second
// Set the LED to blue
setColor(255, 255, 0); // Blue ON, Red and Green OFF
delay(1000); // Wait for 1 second
// Set the LED to yellow
setColor(0, 0, 255); // Red and Green ON, Blue OFF
delay(1000); // Wait for 1 second
// Set the LED to cyan
setColor(255, 0, 0); // Green and Blue ON, Red OFF
delay(1000); // Wait for 1 second
// Set the LED to magenta
setColor(0, 255, 0); // Red and Blue ON, Green OFF
delay(1000); // Wait for 1 second
// Turn off the LED
setColor(255, 255, 255);
delay(1000); // Wait for 1 second
}
// Function to set the color of the RGB LED
void setColor(int redValue, int greenValue, int blueValue) {
analogWrite(redPin, redValue);
analogWrite(greenPin, greenValue);
analogWrite(bluePin, blueValue);
}
Explanation of the Code
- Pin Definitions: The pins for the red, green, and blue LEDs are defined.
- Setup Function: Sets the RGB LED pins as outputs.
- Loop Function: Changes the color of the RGB LED by calling the
setColor()
function with different values. - setColor() Function: Uses the
analogWrite()
function to set the brightness of each LED.
Testing and Troubleshooting
- Check Connections: Ensure the connections
- Adjust Colors: You can fine-tune the RGB values in
setColor()
for different colors.
Arduino Projects:
Arduino Project 1 – Interactive RGB LED Color Controller Using Push Buttons
In this project, we use a push button to control an RGB LED with three distinct actions: a single press changes the LED color, a double press triggers a smooth fading effect, and a long press turns the LED off. The LED cycles through various colors, including red, green, blue, and more, with the added feature of a breathing effect for a dynamic display. By leveraging the simplicity of an Arduino, this project offers an engaging and interactive way to control RGB lighting with minimal hardware. This project is perfect for beginners looking to explore basic input-output operations and LED control.
Required Components
- Arduino UNO R3 SMD
- KY-016 RGB LED module
- Push button
- Jumper wires
- Breadboard
Circuit Diagram / Wiring
- RGB with Arduino
- RGB (GND) → Arduino GND
- RGB (RED) → Arduino D9
- RGB (GREEN) → Arduino D10
- RGB (BLUE) → Arduino D11
- Push Button with Arduino
- BUTTON (VCC) → Arduino 5V,3V
- BUTTON (GND) → Arduino GND
- BUTTON (SINGNAL) → Arduino D8
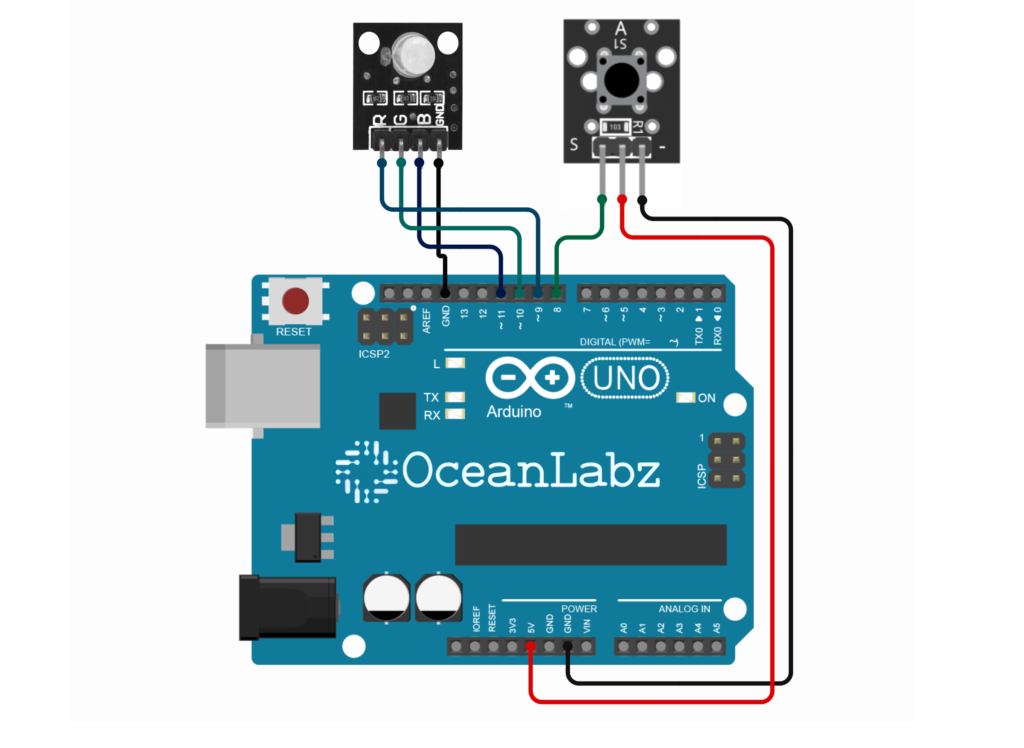
Arduino Code
#define BUTTON_PIN 8
#define RED_PIN 9
#define GREEN_PIN 10
#define BLUE_PIN 11
unsigned long lastPressTime = 0;
unsigned long pressDuration = 0;
unsigned long debounceTime = 200; // Debounce delay
unsigned long doublePressInterval = 500; // Max time between double presses
unsigned long longPressDuration = 2000; // Minimum time for a long press
bool fadeEffect = false; // Flag for fade effect
int colorIndex = 0; // Tracks current static color index
// Define static colors (R, G, B)
int colors[][3] = {
{255, 0, 0}, // Red
{0, 255, 0}, // Green
{0, 0, 255}, // Blue
{255, 255, 0}, // Yellow
{0, 255, 255}, // Cyan
{255, 0, 255}, // Magenta
{255, 255, 255} // White
};
void setup() {
pinMode(BUTTON_PIN, INPUT_PULLUP); // Push switch module signal pin
pinMode(RED_PIN, OUTPUT);
pinMode(GREEN_PIN, OUTPUT);
pinMode(BLUE_PIN, OUTPUT);
setColor(0, 0, 0); // Turn off LED initially
}
void loop() {
static bool lastButtonState = HIGH;
bool buttonState = digitalRead(BUTTON_PIN);
// Track press duration
if (buttonState == LOW) {
pressDuration = millis() - lastPressTime;
} else if (lastButtonState == LOW && buttonState == HIGH) {
// Button released
if (pressDuration > longPressDuration) {
// Long press detected
fadeEffect = false; // Stop fade effect
setColor(0, 0, 0); // Turn off LED
Serial.println("Long Press: LED Off");
} else if (pressDuration < longPressDuration && pressDuration > debounceTime) {
unsigned long currentTime = millis();
if (currentTime - lastPressTime < doublePressInterval && !fadeEffect) {
// Double press detected
fadeEffect = true; // Start fade effect
Serial.println("Double Press: Fade Effect On");
} else if (fadeEffect) {
// Toggle fade effect off
fadeEffect = false;
Serial.println("Double Press: Fade Effect Off");
} else {
// Single press detected
colorIndex = (colorIndex + 1) % (sizeof(colors) / sizeof(colors[0])); // Cycle through colors
setColor(colors[colorIndex][0], colors[colorIndex][1], colors[colorIndex][2]);
Serial.println("Single Press: Change Color");
}
}
lastPressTime = millis(); // Update last press time
pressDuration = 0; // Reset press duration
}
lastButtonState = buttonState;
// Handle fade effect
if (fadeEffect) {
fadeColors();
}
}
// Function to fade colors (breathing effect)
void fadeColors() {
for (int i = 0; i <= 255; i++) { // Fade up
setColor(i, 0, 255 - i); // Purple to Blue
delay(5);
}
for (int i = 255; i >= 0; i--) { // Fade down
setColor(i, 255 - i, 0); // Blue to Green
delay(5);
}
for (int i = 0; i <= 255; i++) { // Fade up
setColor(255 - i, i, 0); // Green to Red
delay(5);
}
for (int i = 255; i >= 0; i--) { // Fade down
setColor(0, i, 255 - i); // Red to Purple
delay(5);
}
}
// Function to set RGB LED module color
void setColor(int red, int green, int blue) {
analogWrite(RED_PIN, red);
analogWrite(GREEN_PIN, green);
analogWrite(BLUE_PIN, blue);
}
Explanation
- Single Press: Cycles through static colors (Red, Green, Blue, Yellow, Cyan, Magenta, White).
- Double Press: Toggles the fade breathing color effect.
- Long Press (Hold for 2 seconds): Turns off the RGB LED.
- Fade Effect: Creates a smooth transition between colors in a breathing pattern (Purple → Blue → Green → Red → Purple).