Index

Introduction
The 28BYJ-48 is a small stepper motor that is easy to control using an Arduino and the ULN2003 driver. This tutorial will guide you through the wiring and programming needed to control the motor.
Required Components
- Arduino UNO or Nano
- 28BYJ-48 stepper motor
- ULN2003 driver board
- Jumper wires
- Breadboard (optional)
Pinout
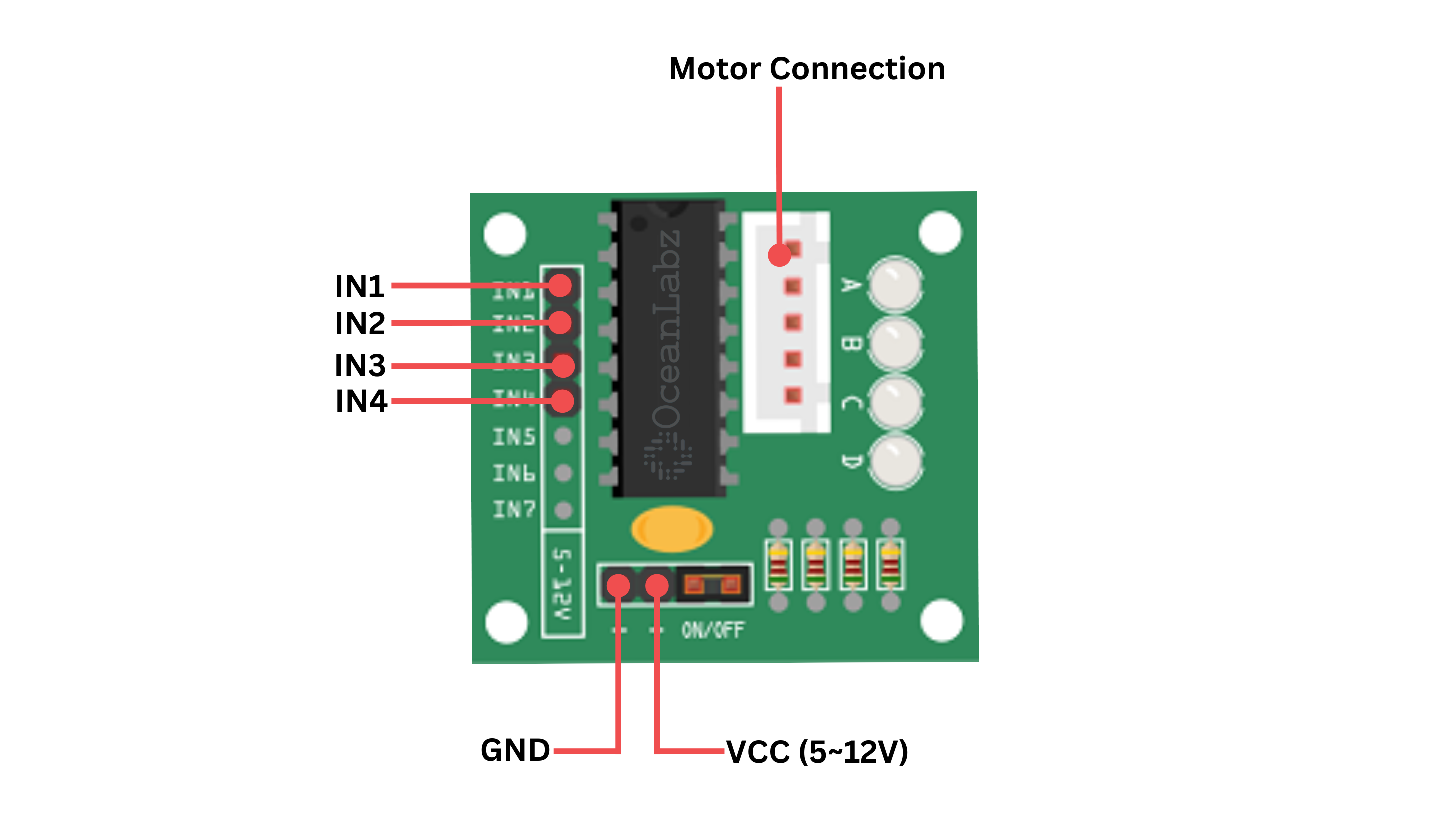
Circuit Diagram / Wiring
- ULN2003 VCC → 5V (Arduino)
- ULN2003 GND → GND (Arduino)
- ULN2003 IN1 → Pin D8 (Arduino)
- ULN2003 IN2 → Pin D9 (Arduino)
- ULN2003 IN3 → Pin D10 (Arduino)
- ULN2003 IN4 → Pin D11 (Arduino)

Arduino Code / Programming
Use the following code to control the 28BYJ-48 stepper motor:
#define IN1 8
#define IN2 9
#define IN3 10
#define IN4 11
void setup() {
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
}
void loop() {
// Rotate clockwise
for (int i = 0; i < 512; i++) {
stepMotor(1);
}
delay(1000); // Wait for 1 second
// Rotate counterclockwise
for (int i = 0; i < 512; i++) {
stepMotor(-1);
}
delay(1000); // Wait for 1 second
}
void stepMotor(int step) {
if (step == 1) { // Clockwise
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
delay(2);
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
delay(2);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
delay(2);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
delay(2);
digitalWrite(IN4, LOW);
digitalWrite(IN1, HIGH);
delay(2);
} else if (step == -1) { // Counterclockwise
digitalWrite(IN4, HIGH);
digitalWrite(IN3, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN1, LOW);
delay(2);
digitalWrite(IN4, LOW);
digitalWrite(IN3, HIGH);
delay(2);
digitalWrite(IN3, LOW);
digitalWrite(IN2, HIGH);
delay(2);
digitalWrite(IN2, LOW);
digitalWrite(IN1, HIGH);
delay(2);
digitalWrite(IN1, LOW);
digitalWrite(IN4, HIGH);
delay(2);
}
}
Explanation of the Code
- Pin Definitions: Define the pins connected to the ULN2003 driver.
- Setup Function: Set the defined pins as outputs.
- Loop Function: The motor rotates 512 steps clockwise, pauses for one second, then rotates 512 steps counterclockwise.
- Step Motor Function: This function controls the motor by activating the appropriate pins in sequence.
Challenges
Challenge 1: Joystick-Controlled Etch-A-Sketch
Problem Statement:
- Use a joystick to control the stepper motor, treating it like an Etch-A-Sketch drawing tool.
- Moving the joystick up or down rotates the motor clockwise or counterclockwise, and moving the joystick left or right speeds up or slows down the rotation.
- The user tries to make patterns by combining joystick movements to control the motor’s position and speed.
Code:
#include <Stepper.h>
const int stepsPerRevolution = 2048;
Stepper myStepper(stepsPerRevolution, 8, 10, 9, 11);
const int xAxisPin = A0;
const int yAxisPin = A1;
int xVal, yVal;
void setup() {
Serial.begin(9600);
myStepper.setSpeed(5);
}
void loop() {
xVal = analogRead(xAxisPin);
yVal = analogRead(yAxisPin);
int speed = map(xVal, 0, 1023, 1, 15);
myStepper.setSpeed(speed);
if (yVal < 400) {
myStepper.step(10);
} else if (yVal > 600) {
myStepper.step(-10);
}
}
Challenge 2: Potentiometer-Controlled Precision Positioning
Problem Statement:
- Use a potentiometer to precisely control the motor’s rotation angle, turning it into a precision positioning tool.
- As the potentiometer is turned, the motor rotates between 0 and 180 degrees.
- This is useful for applications where specific angles are required and lets users fine-tune motor positioning.
Code:
#include <Stepper.h>
const int stepsPerRevolution = 2048;
Stepper myStepper(stepsPerRevolution, 8, 10, 9, 11);
const int potPin = A0;
int potValue, lastPosition = 0;
void setup() {
Serial.begin(9600);
myStepper.setSpeed(10);
}
void loop() {
potValue = analogRead(potPin);
int targetPosition = map(potValue, 0, 1023, 0, stepsPerRevolution / 2);
int stepsToMove = targetPosition - lastPosition;
myStepper.step(stepsToMove);
lastPosition = targetPosition;
}
Testing and Troubleshooting
- Check Connections: Ensure all connections are secure.
- Power Supply: Make sure the motor is powered by the Arduino or an external power source.
- Adjust Delays: You can modify the delay in the
stepMotor
function to change the speed of rotation.