What are Pointers ?
A pointer is a variable that holds the memory address of another variable. For example, in the below gif we can see that a is variable that holds the value 10 and has a memory address 1100, whereas p is another variable that holds the address of a. so here in this example p is a pointer variable that is pointing on a.
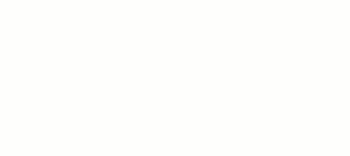
Let’s understand the concept of pointer in 5 Quick steps
Step 1) Pointer syntax
This is how we declare pointer
<data_type> <*variable_name>; //pointer variable declaration
<data_type> <variable_name>; //normal variable declaration
where data_type is the valid C data type and variable_name is a name of pointer variable
Take a look at few more valid pointer declarations
int *p; //pointer to an integer
char *p; //pointer to a character
float *p; //pointer to a float
Step 2) To get the address ( & )
As we already know that all the variables defines in a program (including pointer variables) located at a specific address and using &(ampersand) it is possible to obtain the address of variable For example,
int john=10;
int *y;
y = &john; //address of john copied to y

john and y are two variables, john of type int whereas y is a integer pointer, the above statement copied the memory address of john into y using & operator. &(ampersand) operator has nothing to do with the value of john. you can think & as returning “the address of”.
Step3) Get the value Pointed by Pointer(* Dereferencing operator)
So far we have learnt how to declare pointer variable and how to get the address of other variable, now its time to get the value of things pointed by pointer, for which we’ll use * operator, let’s take a look below.
int john=10;
int *ptr;
ptr = &john;
printf("%d", *ptr);
we already have discussed the above three lines in step 2, using the * dereferencing operator before the pointer name gives rights to access and manipulate data containing in the memory location pointed by a pointer, so to get and change the value of john we need to use *ptr
Lets take a quick example to change the value of john using pointer
#include <stdio.h>
int main()
{
int john=10;
int *ptr;
ptr = &john;
printf("%d\n", *ptr);
*ptr = 20;
printf("%d\n",*ptr);
}
output
10
20
Let’s take one more example.
int *ptr;
int a=10, b=20;
ptr=&a;
printf("value of a = %d", *ptr);
ptr=&b;
printf("value of b = %d, *ptr");
output
value of a = 10
value of b = 20
lets understand this code line by line
line 1
int john=10;

A variable name john is created with an initial value of 10. let’s assume the address of variable 11211.
line 2
int *ptr;
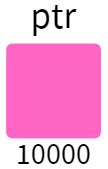
a pointer to integer is created. let’s assume the address 10000.
line 3
printf("address of john=%d",&john);
address of john is 11211.
line 4
printf("value of john=%d",john);
value of john is 10.
line 5
ptr=&john;
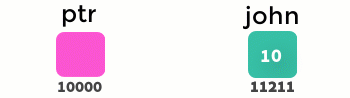
ptr pointer is now pointing to john and holding a address of john.
line 6
printf("address hold by pointer=%d",&ptr);
address of ptr is 10000.
line 7
printf("value of ptr is=%d",*ptr);
(*) before ptr gives the value of variable at which pointer is pointing that is 10.
line 8
john=15;
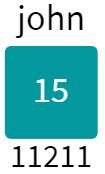
assign value 15 to john.
line 9
printf("address hold by pointer=%d,&ptr");
nothing has changed in the address of ptr.
line 10
printf("value of ptr is=%d",*ptr);
(*) before ptr gives the value of variable at which pointer is pointing, value of john has now changed to 15, so *ptr is now become 15.
line 11
*ptr=0;

as we have discussed earlier that * before pointer name gives the right to manipulate the value of variable at which pointer is pointing, pointer is pointing to john therefore the value of john become now 0.
line 12
printf("address of john=%d",&john);
address of john remain same i.e. 11211.
line 13
printf("value of john=%d",john);
value of john now has become 0.