Index
Imagine a TV remote with different buttons, and each button performs a specific action (like changing channels, adjusting volume, or powering off the TV). Using a switch-case
statement, you can simulate the behavior of a TV remote where pressing a certain button triggers a specific response. For better understanding see the short video below
The switch
statement in C is a control structure used to execute one of many code blocks based on the value of an expression. It’s particularly useful when there are multiple conditions to check, as it provides a cleaner and more efficient alternative to multiple if-else
statements.
How It Works
- The
switch
statement evaluates an expression, usually a variable. - Based on the result, it jumps to the corresponding
case
label. - If a match is found, it executes the code under that
case
. - The
break
statement is used to exit theswitch
structure once the matchedcase
block is complete. - If none of the cases match, the
default
block (optional) executes as a fallback.
Syntax
switch (expression) {
case constant1:
// code block 1
break;
case constant2:
// code block 2
break;
// add more cases as needed
default:
// code block for default case
break;
}
expression
: This is the value that theswitch
statement evaluates. It can be of integral type (char, int, long) or an enumeration.case constant1
,case constant2
, …: These are the possible values that the expression can take. If the expression matches one of these constants, the corresponding code block following that case label will be executed.break
: Thebreak
statement is used to terminate theswitch
statement and exit the switch block. Ifbreak
is omitted, control will fall through to the next case.default
: This is an optional case label that is used when none of the other cases match the value of the expression. It’s similar to theelse
clause in anif-else
statement.
Flowchart:
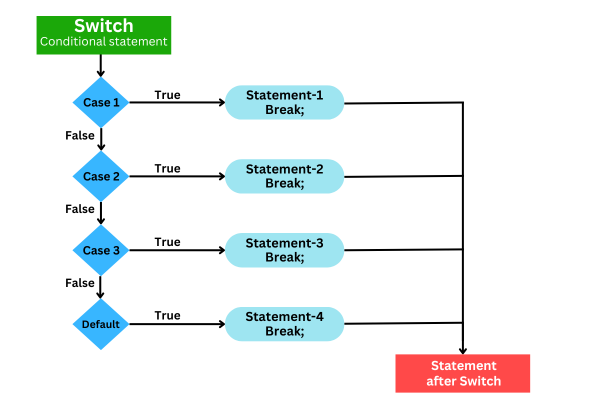
Here’s an example of using the switch
statement:
Example 1: Enter Keyword for a choice
#include <stdio.h>
int main() {
int choice = 2;
switch (choice) {
case 1:
printf("You chose option 1\n");
break;
case 2:
printf("You chose option 2\n");
break;
case 3:
printf("You chose option 3\n");
break;
default:
printf("Invalid choice\n");
break;
}
return 0;
}
Output:
You chose option 2
Explanation:
- The
switch
keyword evaluates the value ofchoice
. Based on its value, it will match one of thecase
labels. - Case 1: If
choice == 1
, the program will execute the code inside this block (printf("You chose option 1\n");
) and then exit the switch using thebreak
statement. - Case 2: If
choice == 2
(which it is in this case), the program will execute the code in this block, printing “You chose option 2” to the console, and then exit the switch withbreak
. - Case 3: If
choice == 3
, it will print “You chose option 3”. - Default Case: If none of the
case
values match, the program will execute thedefault
block and print “Invalid choice”. - The
break
statement prevents the program from continuing to check the next cases after the matching case is executed. Without thebreak
, the program would execute all the code blocks after the matched case, even if they don’t match (this is called “fall-through”).
Example 2: Number to Day Conversion
#include <stdio.h>
int main() {
int day;
printf("Enter a number (1-7) to get the corresponding day of the week: ");
scanf("%d", &day);
switch(day) {
case 1:
printf("Sunday\n");
break;
case 2:
printf("Monday\n");
break;
case 3:
printf("Tuesday\n");
break;
case 4:
printf("Wednesday\n");
break;
case 5:
printf("Thursday\n");
break;
case 6:
printf("Friday\n");
break;
case 7:
printf("Saturday\n");
break;
default:
printf("Invalid day number. Please enter a number between 1 and 7.\n");
}
return 0;
}
Explanation
- Expression:
day
is the expression evaluated in theswitch
statement. - Cases: Each
case
label represents a possible value forday
. When a match is found, the corresponding code executes. - Break Statements: The
break
statement prevents the execution from falling through to subsequent cases, ensuring only the matched case executes. - Default Case: The
default
block is executed if none of the cases match. Here, it catches invalid inputs.
Use Cases and Advantages of switch
- Menu-driven Programs: When building programs with menu options,
switch
can efficiently handle the selection logic. - Improved Readability: Using
switch
instead ofif-else
for multi-option selections makes the code more readable and structured. - Efficiency: The
switch
statement may be more efficient than multipleif-else
checks, depending on the compiler.